The simplest way to search for news articles
How to quickly start using the Python SDK to search for articles. Use the iterators to quickly iterate over matching articles.
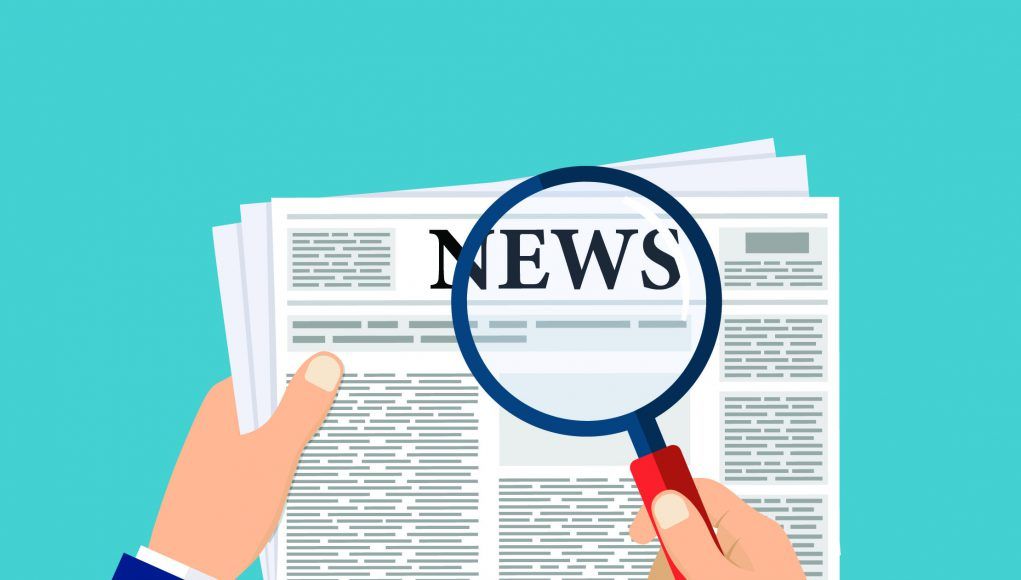
The most common task that our users perform is searching for news articles. In this post, we will demonstrate how to use our news searching API with the Python SDK and how to retrieve as many results as you'd like with the least amount of code.
If you don't yet have our eventregistry
package installed, install it using:
>>>pip install eventregistry
If you don't have your API key, visit the registration page and create a free account. After you activate it, go to your dashboard, where you will find the API key, which you'll need when creating the EventRegistry
class instance.
from eventregistry import *
er = EventRegistry(apiKey = YOUR_API_KEY)
EventRegistry
instance that is used to communicate with our servicesAs a simple example, let's assume that you want to find news about Tesla. In that case, you can use the following code:
# choose how many articles at most you want to download
MAX_RESULTS = 500
# create the query with all conditions that we want to search for
q = QueryArticlesIter(keywords = "Tesla Inc")
# execute the search and process article by article
for art in q.execQuery(er, maxItems = MAX_RESULTS):
# do something with the retrieved article
print(art)
The QueryArticlesIter
class allows you to specify your search conditions. In this case, we want to find articles that simply mention the phrase "Tesla Inc".
The nice thing about the QueryArticlesIter
class is, that it can serve as an iterator - it can return you as many results as there are available. The class still downloads the articles in batches of 100, but will automatically download you next 100 articles each time you use the already-downloaded articles in the for
loop.
Keep in mind that each time the iterator will download you next 100 articles, the appropriate number of tokens will be used from your account. If you wish to avoid mistakenly downloading a huge number of articles, make sure you specify the maxItems
parameter when calling the execQuery()
method.
Note that you can also search for articles using many other filters. Examples of these other filters include:
- concepts - entities and things that we are able to recognize and disambiguate in the articles,
- categories - article topics, such as sports, investment, natural disasters, etc.,
- sources - source that published the news article,
- source locations - the location of the news source (e.g. get articles published by sources located in NY or Germany),
- authors - who is the person that wrote the article,
- language - get only content published in a particular language,
- date - find only articles published in a particular date range,
- sentiment - get only articles with a particular sentiment
To see the full list of available filters please check our GitHub documentation page.